SQL Injection
SQL Injection
SQL Injection (SQLi) is one of the most common and dangerous vulnerabilities in the field of cybersecurity. This type of attack allows malicious actors to inject harmful SQL code into an application, granting unauthorized access to databases or manipulating their contents. If undetected and unmitigated, SQL Injection can lead to severe consequences, including data theft, database corruption, and damage to an organization's reputation.
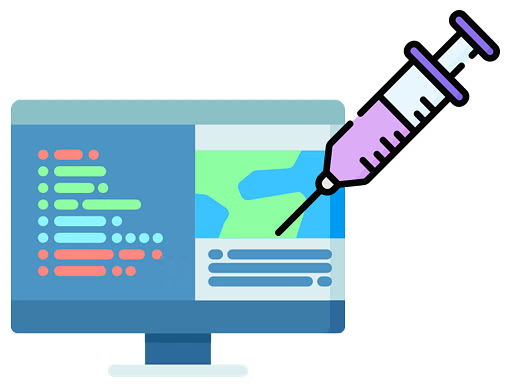
How Does SQL Injection Work?
Most applications use SQL (Structured Query Language) to communicate with their databases. The issue arises when an application improperly handles user input. Consider a simple login form:
SELECT * FROM users WHERE username = 'user' AND password = 'password';
An attacker could input the following malicious string into the password field:
' OR '1'='1
This alters the query to:
SELECT * FROM users WHERE username = 'user' AND password = '' OR '1'='1';
Since the condition '1'='1' is always true, the database might authenticate the attacker without needing valid credentials.
Real-World Implications of SQL Injection
SQL Injection attacks can have devastating effects, including:
Unauthorized Access: Hackers can access sensitive data such as usernames, passwords, or financial information.
Data Manipulation: Attackers can modify or delete data, causing business disruption.
Exploitation for Further Attacks: Gaining a foothold through SQLi can enable attackers to launch more sophisticated attacks, such as ransomware or phishing campaigns.
Compliance Violations: Exposing sensitive data could lead to penalties under regulations like GDPR or HIPAA.
Types of SQL Injection
There are several types of SQL Injection attacks:
Classic SQL Injection: Directly injecting malicious SQL code.
Blind SQL Injection: When the attacker cannot see the database output but infers information based on application behavior.
Boolean-Based Blind SQLi: Exploits true/false statements to extract information.
Time-Based Blind SQLi: Delays in server responses help attackers deduce data.
Error-Based SQLi: Leverages database error messages to retrieve information.
How to Protect Against SQL Injection
Use Prepared Statements and Parameterized Queries:
Avoid dynamic queries. Use placeholders for user input.
Example in Python with sqlite:
cursor.execute("SELECT * FROM users WHERE username = ? AND password = ?", (username, password))
Validate and Sanitize User Input:
Ensure inputs conform to expected formats (e.g., email, numbers).
Strip harmful characters or patterns.
Implement Proper Error Handling:
Avoid displaying detailed error messages to users. Use generic error messages.
Use ORM Frameworks:
Frameworks like Django, Hibernate, or Entity Framework abstract SQL queries, reducing the risk of SQL Injection.
Regular Security Testing:
Perform penetration testing and vulnerability scans to identify and fix weaknesses.
Restrict Database Permissions:
Minimize privileges for database accounts to limit the scope of potential damage.
Web Application Firewalls (WAF):
Deploy WAFs to detect and block malicious queries.
SQL Injection remains a significant threat, but it is preventable with the right precautions. By understanding the risks, implementing secure coding practices, and regularly testing for vulnerabilities, you can safeguard your applications and data from potential exploitation. Investing in security not only protects your business but also builds trust with your users.
That's it, stay safe.
Luka Vukovic
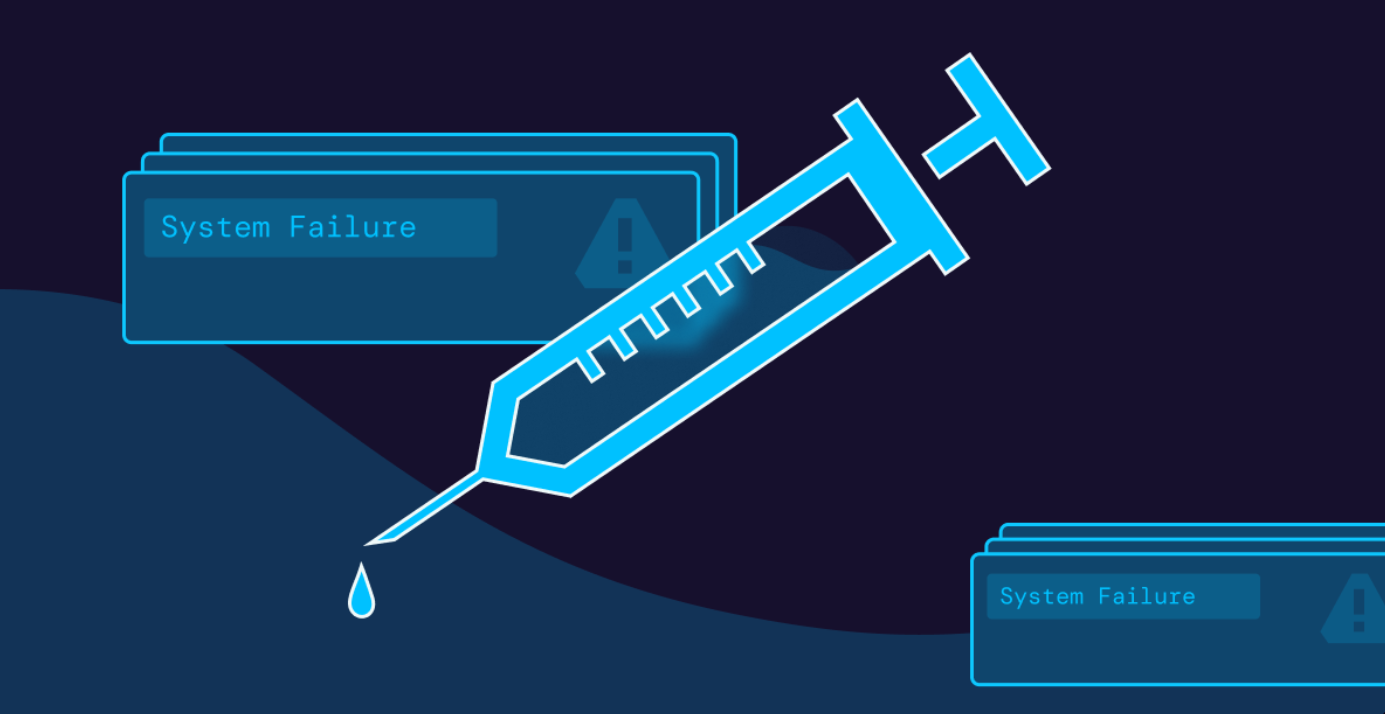