Stack and Heap in Java
Stack and Heap in Java
When working in java its helpful to know what's happening behind the scenes. One of the most important things in java and probably in any other language is memory management. You probably wondered what's happening when you call function or when you make new object or variable. If you have but also if you haven’t this is right place for you because I will explain stack and heap in this post.
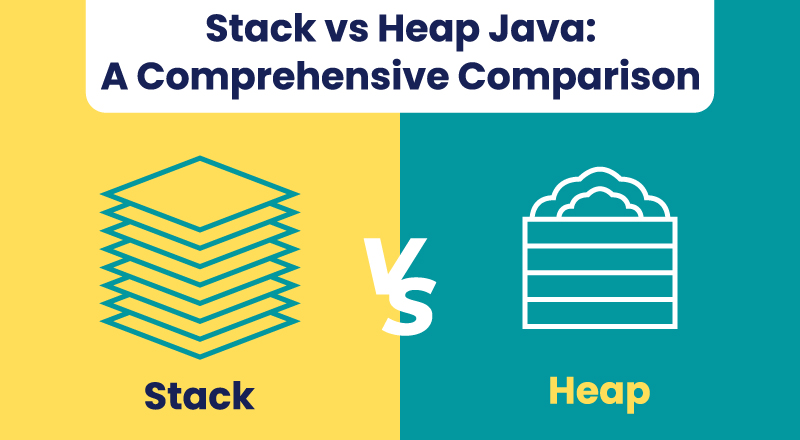
So everything we do in our program needs to be either in stack or heap, that’s clear but what’s going where and how do stack and heap look like. I will use simple code to explain that in practical way so you do not need to imagine that process in your mind. But before that I will just write simple definitions of stack and heap so you can orient in further text.
Stack
Java Stack memory is used for the execution of a thread. Every method has its own stack which its short lived or in other words last as long as that method. After method is done with executing that memory is released and free for other things. A stack is a data structure that follows the Last In, First Out (LIFO) principle, meaning that the last element added to the stack will be the first one to be removed. Stack memory is much smaller than heap.
Heap
Java Heap space is used by java runtime to allocate memory to Objects and JRE classes. Whenever we create an object, it’s always created in the Heap space. General purpose and idea of heap is to have space to store objects and data that will live longer than the method or function that created them.
What It Does:
• The heap is used to store objects created during program execution, such as strings, arrays, or any instance of a class.
• Unlike temporary variables (stored in the stack), data in the heap can be shared and accessed by different parts of the program.
Key Features:
• Objects in the heap stay in memory as long as they are needed (until the program is done using them or they become "unreachable").
• The Java Garbage Collector automatically removes unused objects from the heap to free up space.
Simplified View:
• Stack: Fast, temporary, local.
• Heap: Slower, shared, long-term storage.
Practical example:
We will look at this program and describe what happens behind the scenes:
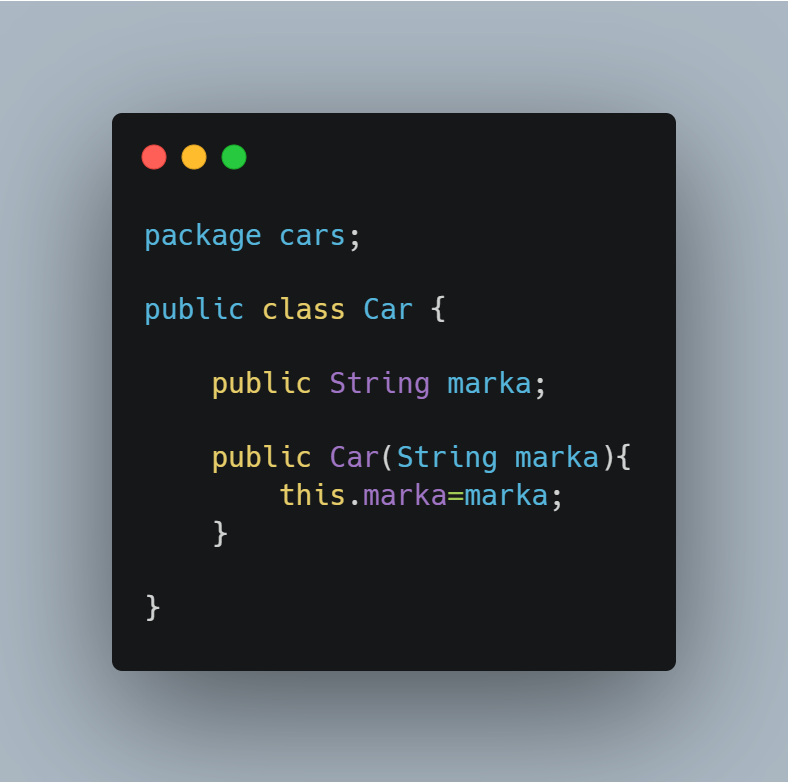
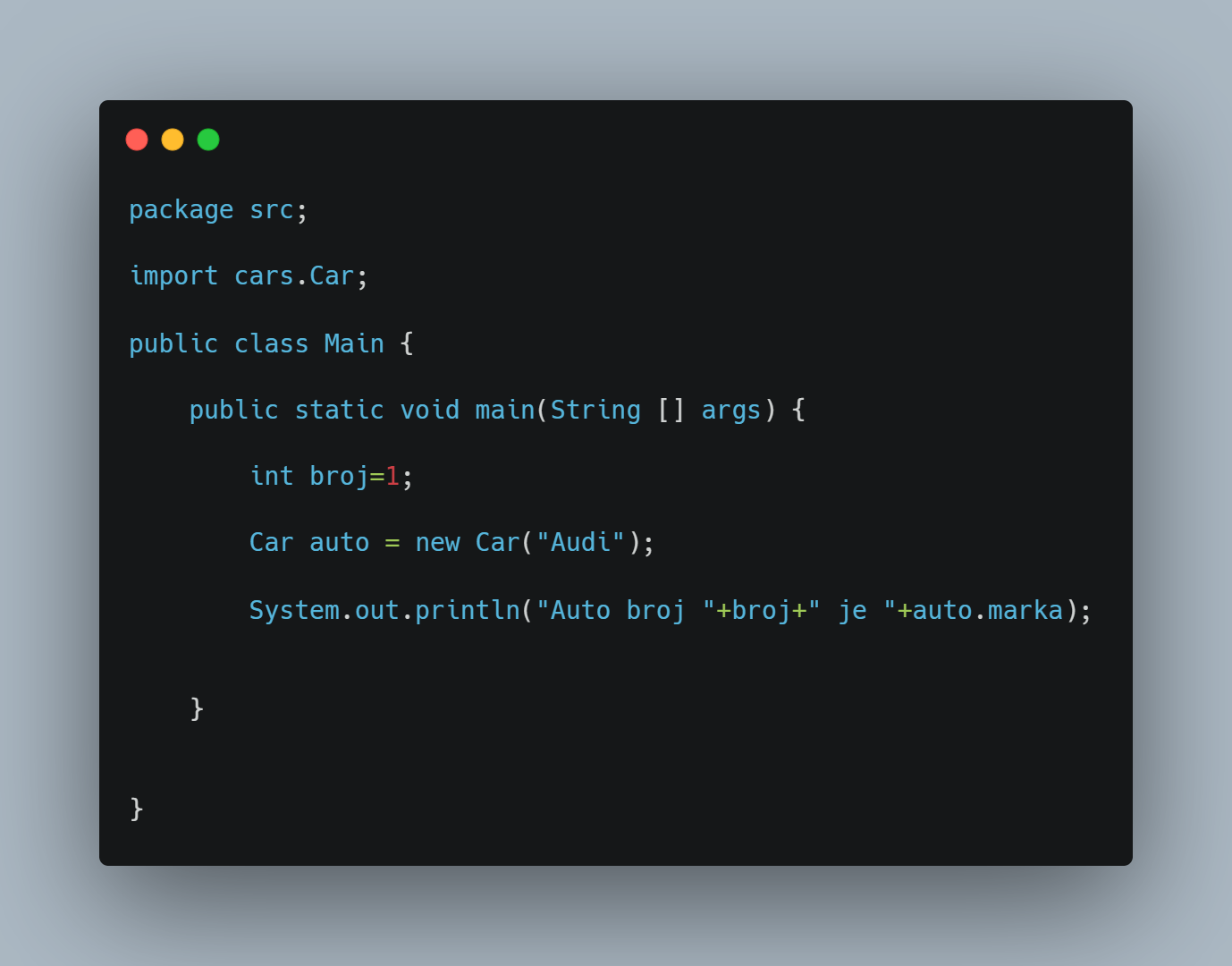
As soon as we run the program, it loads all the Runtime classes into the Heap space. When the main() method is found at line 1, Java Runtime creates stack memory to be used by main() method thread.
The args parameter (an array of String) is created on the stack memory, pointing to an object in the heap if arguments are passed to the program.
Second thing is declaring int variable broj and giving it value 1, at that point we have block at stack for everything inside main method so we add variable broj and its value 1.
After that we initialize object auto of type Car. Do you have any idea whats happening with that, knowing basic definitions of stack and heap what is going where.
At first it’s maybe frustrating thinking about that but its very simple and easy method that will always be in your head after you understand it well.
So where were we. Object auto will take its space in heap memory and also have reference in main stack block, so in stack, auto will have value of memory address of object from heap. If you know pointers from c or other low level language this is probably easy for you to understand but if you are not familiar with that check this Also Its field marka is initialized with the value "Audi".
What’s happening with print statement?
The program accesses:
1. The value of broj from the stack memory.
2. The marka field of the Car object in the heap memory, using the reference auto from the stack.
The string concatenation happens in memory (likely using temporary stack space), and the final string "Auto broj 1 je Audi" is passed to the System.out.println() method. The println() method uses the standard output stream to display the string.
So basically that’s it, read it few times and try some of your own examples and you’ll be fine.
Luka Vukovic